根据JSON生成JavaBean
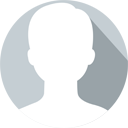
admin 发布于:2017-02-16 21:50:39
阅读:loading
根据json格式数据生成一个javabean对象,首先这是一个简单的功能,但是要写出一个比较好的版本实际还是有些难点的,这里跟大家分享一种实现,基于fastjson、freemarker。
1. json格式中可包括boolean类型、String类型、int类型、金额类型等常见类型;
2. json格式中可出现其它关联对象,包括其对象类型、集合类型;
3. 相关联的对象类不能作为一个单独的类创建,作为子类创建在内部;
4. 理论上支持无限极JSON对象格式(可能会出现子类、孙子类、重孙子类等);
1.json文本
{
"active": true,
"date": "2016-12-20",
"id": 1001,
"money": 5.25,
"name": "china"
}
2.生成的Java类
package com.vo;
import java.math.BigDecimal;
/**
* <pre>
*
* </pre>
*
* @auth chendd
* @date 2017-02-16
*/
public class User1 {
private Integer id;
private String name;
private BigDecimal money;
private Boolean active;
private String date;
/**
* @return id
*/
public Integer getId() {
return id;
}
/**
* @param id
*/
public void setId(Integer id) {
this.id = id;
}
/**
* @return name
*/
public String getName() {
return name;
}
/**
* @param name
*/
public void setName(String name) {
this.name = name;
}
/**
* @return money
*/
public BigDecimal getMoney() {
return money;
}
/**
* @param money
*/
public void setMoney(BigDecimal money) {
this.money = money;
}
/**
* @return active
*/
public Boolean getActive() {
return active;
}
/**
* @param active
*/
public void setActive(Boolean active) {
this.active = active;
}
/**
* @return date
*/
public String getDate() {
return date;
}
/**
* @param date
*/
public void setDate(String date) {
this.date = date;
}
}
1.json文本
{
"active": true,
"date": "2016-12-20",
"id": 1001,
"employee": {
"empId": 10086,
"empName": "zhang3",
"money": 5.25
},
"name": "china"
}
2.生成的Java类
package com.vo;
import java.math.BigDecimal;
/**
* <pre>
*
* </pre>
*
* @auth chendd
* @date 2017-02-16
*/
public class User2 {
private Integer id;
private String name;
private Boolean active;
private String date;
private Employee employee;
public static class Employee {
//dg
private String empName;
/**
* @return empName
*/
public String getEmpName() {
return this.empName;
}
/**
* @param empName
*/
public void setEmpName(String empName) {
this.empName = empName;
}
private BigDecimal money;
/**
* @return money
*/
public BigDecimal getMoney() {
return this.money;
}
/**
* @param money
*/
public void setMoney(BigDecimal money) {
this.money = money;
}
private Integer empId;
/**
* @return empId
*/
public Integer getEmpId() {
return this.empId;
}
/**
* @param empId
*/
public void setEmpId(Integer empId) {
this.empId = empId;
}
}
/**
* @return id
*/
public Integer getId() {
return id;
}
/**
* @param id
*/
public void setId(Integer id) {
this.id = id;
}
/**
* @return name
*/
public String getName() {
return name;
}
/**
* @param name
*/
public void setName(String name) {
this.name = name;
}
/**
* @return active
*/
public Boolean getActive() {
return active;
}
/**
* @param active
*/
public void setActive(Boolean active) {
this.active = active;
}
/**
* @return employee
*/
public Employee getEmployee() {
return employee;
}
/**
* @param employee
*/
public void setEmployee(Employee employee) {
this.employee = employee;
}
/**
* @return date
*/
public String getDate() {
return date;
}
/**
* @param date
*/
public void setDate(String date) {
this.date = date;
}
}
1.json文本
{
"active": true,
"date": "2016-12-20",
"id": 1001,
"employee": {
"empId": 10086,
"empName": "zhang3",
"money": 5.25
},
"name": "china",
"accountList": [
{"accId" : 1 , "accName": "lisi" , sex: "男"},
{"accId" : 2 , "accName": "chen2" , sex: "女"}
]
}
2.生成的Java类
package com.vo;
import java.util.*;
import java.math.BigDecimal;
/**
* <pre>
*
* </pre>
*
* @auth chendd
* @date 2017-02-16
*/
public class User3 {
private Integer id;
private String name;
private Boolean active;
private String date;
private List<Account> accountList;
private Employee employee;
public static class Account {
//dg
private String sex;
/**
* @return sex
*/
public String getSex() {
return this.sex;
}
/**
* @param sex
*/
public void setSex(String sex) {
this.sex = sex;
}
private String accName;
/**
* @return accName
*/
public String getAccName() {
return this.accName;
}
/**
* @param accName
*/
public void setAccName(String accName) {
this.accName = accName;
}
private Integer accId;
/**
* @return accId
*/
public Integer getAccId() {
return this.accId;
}
/**
* @param accId
*/
public void setAccId(Integer accId) {
this.accId = accId;
}
}
public static class Employee {
//dg
private String empName;
/**
* @return empName
*/
public String getEmpName() {
return this.empName;
}
/**
* @param empName
*/
public void setEmpName(String empName) {
this.empName = empName;
}
private BigDecimal money;
/**
* @return money
*/
public BigDecimal getMoney() {
return this.money;
}
/**
* @param money
*/
public void setMoney(BigDecimal money) {
this.money = money;
}
private Integer empId;
/**
* @return empId
*/
public Integer getEmpId() {
return this.empId;
}
/**
* @param empId
*/
public void setEmpId(Integer empId) {
this.empId = empId;
}
}
/**
* @return id
*/
public Integer getId() {
return id;
}
/**
* @param id
*/
public void setId(Integer id) {
this.id = id;
}
/**
* @return accountList
*/
public List<Account> getAccountList() {
return accountList;
}
/**
* @param accountList
*/
public void setAccountList(List<Account> accountList) {
this.accountList = accountList;
}
/**
* @return name
*/
public String getName() {
return name;
}
/**
* @param name
*/
public void setName(String name) {
this.name = name;
}
/**
* @return active
*/
public Boolean getActive() {
return active;
}
/**
* @param active
*/
public void setActive(Boolean active) {
this.active = active;
}
/**
* @return employee
*/
public Employee getEmployee() {
return employee;
}
/**
* @param employee
*/
public void setEmployee(Employee employee) {
this.employee = employee;
}
/**
* @return date
*/
public String getDate() {
return date;
}
/**
* @param date
*/
public void setDate(String date) {
this.date = date;
}
}
1.json文本
{
"active": true,
"date": "2016-12-20",
"id": 1001,
"employee": {
"empId": 10086,
"empName": "zhang3",
"emp11": {
"a1": "a1",
"a2": "a2",
"aa_11": {
"aa_11_aa":"111",
"aa_11_bb":"222"
},
"a3": "a3"
},
"money": 5.25
},
"name": "china",
"accountList": [
{"accId" : 1 , "accName": "lisi" , sex: "男",
"account11": {
"acc1": 100,
"acc2": "name",
"bbbList": [{
"b1": 1,
"b2": "bbb",
"b3": "bb33"
}],
"acc3": "name"
}
},
{"accId" : 2 , "accName": "chen2" , sex: "女"}
]
}
2.生成的Java类
package com.vo;
import java.util.*;
import java.math.BigDecimal;
/**
* <pre>
*
* </pre>
*
* @auth chendd
* @date 2017-02-16
*/
public class User4 {
private Integer id;
private String name;
private Boolean active;
private String date;
private List<Account> accountList;
private Employee employee;
public static class Account {
//dg
private String sex;
/**
* @return sex
*/
public String getSex() {
return this.sex;
}
/**
* @param sex
*/
public void setSex(String sex) {
this.sex = sex;
}
private String accName;
/**
* @return accName
*/
public String getAccName() {
return this.accName;
}
/**
* @param accName
*/
public void setAccName(String accName) {
this.accName = accName;
}
private Account11 account11;
public Account11 getAccount11() {
return this.account11;
}
public void setAccount11(Account11 account11) {
this.account11 = account11;
}
public static class Account11 {
private String acc2;
/**
* @return acc2
*/
public String getAcc2() {
return this.acc2;
}
/**
* @param acc2
*/
public void setAcc2(String acc2) {
this.acc2 = acc2;
}
private Integer acc1;
/**
* @return acc1
*/
public Integer getAcc1() {
return this.acc1;
}
/**
* @param acc1
*/
public void setAcc1(Integer acc1) {
this.acc1 = acc1;
}
private String acc3;
/**
* @return acc3
*/
public String getAcc3() {
return this.acc3;
}
/**
* @param acc3
*/
public void setAcc3(String acc3) {
this.acc3 = acc3;
}
private List<Bbb> bbbList;
public List<Bbb> getBbbList() {
return this.bbbList;
}
public void setBbbList(List<Bbb> bbbList) {
this.bbbList = bbbList;
}
public static class Bbb {
private Integer b1;
/**
* @return b1
*/
public Integer getB1() {
return this.b1;
}
/**
* @param b1
*/
public void setB1(Integer b1) {
this.b1 = b1;
}
private String b3;
/**
* @return b3
*/
public String getB3() {
return this.b3;
}
/**
* @param b3
*/
public void setB3(String b3) {
this.b3 = b3;
}
private String b2;
/**
* @return b2
*/
public String getB2() {
return this.b2;
}
/**
* @param b2
*/
public void setB2(String b2) {
this.b2 = b2;
}
}
/**
* @return bbbList
*/
}
/**
* @return account11
*/
private Integer accId;
/**
* @return accId
*/
public Integer getAccId() {
return this.accId;
}
/**
* @param accId
*/
public void setAccId(Integer accId) {
this.accId = accId;
}
}
public static class Employee {
//dg
private String empName;
/**
* @return empName
*/
public String getEmpName() {
return this.empName;
}
/**
* @param empName
*/
public void setEmpName(String empName) {
this.empName = empName;
}
private BigDecimal money;
/**
* @return money
*/
public BigDecimal getMoney() {
return this.money;
}
/**
* @param money
*/
public void setMoney(BigDecimal money) {
this.money = money;
}
private Integer empId;
/**
* @return empId
*/
public Integer getEmpId() {
return this.empId;
}
/**
* @param empId
*/
public void setEmpId(Integer empId) {
this.empId = empId;
}
private Emp11 emp11;
public Emp11 getEmp11() {
return this.emp11;
}
public void setEmp11(Emp11 emp11) {
this.emp11 = emp11;
}
public static class Emp11 {
private String a1;
/**
* @return a1
*/
public String getA1() {
return this.a1;
}
/**
* @param a1
*/
public void setA1(String a1) {
this.a1 = a1;
}
private String a2;
/**
* @return a2
*/
public String getA2() {
return this.a2;
}
/**
* @param a2
*/
public void setA2(String a2) {
this.a2 = a2;
}
private String a3;
/**
* @return a3
*/
public String getA3() {
return this.a3;
}
/**
* @param a3
*/
public void setA3(String a3) {
this.a3 = a3;
}
private Aa_11 aa_11;
public Aa_11 getAa_11() {
return this.aa_11;
}
public void setAa_11(Aa_11 aa_11) {
this.aa_11 = aa_11;
}
public static class Aa_11 {
private String aa_11_bb;
/**
* @return aa_11_bb
*/
public String getAa_11_bb() {
return this.aa_11_bb;
}
/**
* @param aa_11_bb
*/
public void setAa_11_bb(String aa_11_bb) {
this.aa_11_bb = aa_11_bb;
}
private String aa_11_aa;
/**
* @return aa_11_aa
*/
public String getAa_11_aa() {
return this.aa_11_aa;
}
/**
* @param aa_11_aa
*/
public void setAa_11_aa(String aa_11_aa) {
this.aa_11_aa = aa_11_aa;
}
}
/**
* @return aa_11
*/
}
/**
* @return emp11
*/
}
/**
* @return id
*/
public Integer getId() {
return id;
}
/**
* @param id
*/
public void setId(Integer id) {
this.id = id;
}
/**
* @return accountList
*/
public List<Account> getAccountList() {
return accountList;
}
/**
* @param accountList
*/
public void setAccountList(List<Account> accountList) {
this.accountList = accountList;
}
/**
* @return name
*/
public String getName() {
return name;
}
/**
* @param name
*/
public void setName(String name) {
this.name = name;
}
/**
* @return active
*/
public Boolean getActive() {
return active;
}
/**
* @param active
*/
public void setActive(Boolean active) {
this.active = active;
}
/**
* @return employee
*/
public Employee getEmployee() {
return employee;
}
/**
* @param employee
*/
public void setEmployee(Employee employee) {
this.employee = employee;
}
/**
* @return date
*/
public String getDate() {
return date;
}
/**
* @param date
*/
public void setDate(String date) {
this.date = date;
}
}
package cn.chendd.test;
import cn.chendd.util.BeanCreateUtil;
import com.alibaba.fastjson.JSONObject;
public class TestJSON {
public static void main(String[] args) throws Exception {
String jsonString = "{\"active\":true,\"date\":\"2016-12-20\",\"id\":1001,\"money\":5,\"name\":\"china\"" +
"," +
" \"emp11\":{\"empId\":1001.25 , \"empName\": \"cdd\" , \"sex\":true , " +
" \"account11\": {\"username\":\"admin\" , \"password\":\"123456\" , \"status\":0} , " +
" \"account22\": {\"username\":\"admin\" , \"password\":\"123456\" , \"status\":0} , " +
"\"accountsList\": [{\"a1\":100 , \"b1\":\"zhangsan\" , \"accBt\" : {\"btId\":100} , \"c1\": true}]" +
" }," +
" \"emp22\":{\"aa2\":1002 , \"bb2\":\"bb2\" , \"cc2\":\"cc2\"} , " +
" \"userList\" : [{ \"userId\": 10086 , \"name\" : \"张三\" , \"city\" : \"湖北\" , " +
" \"uu1\" : {\"uId\":1001 , \"uName\":\"cdd\"}," +
" \"uu2\" : {\"uId\":1001 , \"uName\":\"cdd\"}" +
" }]" +
"}";
JSONObject jsonObject = JSONObject.parseObject(jsonString);
BeanCreateUtil.createFile("com.vo.Employee", jsonObject);
System.out.println("======文件已经创建======");
}
}
点赞