SpringCloud OpenFeign(二)进阶应用
SpringCloud OpenFeign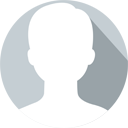
admin 发布于:2023-01-31 10:15:11
阅读:loading
前一篇文章介绍了Open Feign的使用示例,并且包含有非常广泛的知识点,对于实际项目上的应用来讲还是不够的,本篇文章将介绍一下进阶的知识点,来使得Open Feign变得更好用。
#feign 接口配置
feign:
client:
config:
default:
#loggerLevel: BASIC
#建立连接所用的时间,适用于网络状况正常的情况下,两端连接所需要的时间
connectTimeout: 15000
#指建立连接后从服务端读取到可用资源所用的时间
readTimeout: 15000
#按注册的服务配置
chendd-nacos-client:
loggerLevel: BASIC
compression:
request:
enabled: true
min-request-size: 2048
mime-types: text/xml,application/xml,application/json
response:
enabled: true
httpclient:
enabled: true
connection-timeout: 15000
#连接池最大连接数
max-connections: 200
#连接池单个路径的最大连接数
max-connections-per-route: 50
okhttp:
enabled: false
#启用熔断
circuitbreaker:
enabled: true
#设置熔断的超时时间
hystrix:
command:
default:
execution:
isolation:
thread:
timeoutInMilliseconds: 15000
#日志配置
logging:
level:
root: info
cn.chendd.api: debug
(1)loggerLevel可指定默认的日志级别,也可以根据微服务名称进行指定,也可以通过代码指定(下文有);
(2)启用请求压缩,当请求大于2M时开启gzip压缩;
(3)使用httpclient来替代底层http请求客户端(默认使用JDK的HttpURLConnection,不包含连接池);
(4)启用熔断和熔断的超时时间;
package cn.chendd.api.client;
import ;
/**
* Open Feign 特性功能点验证
* @author chendd
* @date 2023/1/14 15:58
*/
@FeignClient(
//微服务名称
name = "chendd-gateway" ,
//url路径,可替换name属性
//url = "",
//接口根路径
path = "/nacos-client/hello" ,
//自定义配置(日志级别、拦截器、url路径覆盖)
configuration = FeatiresFeignConfiguration.class ,
//错误处理器,推荐使用fallbackFactory,fallback无法获取到异常堆栈
//fallback = OpenFeignFeaturesClientFallback.class
fallbackFactory = OpenFeignFeaturesClientFallbackFactory.class
)
public interface OpenFeignFeaturesClient {
/**
* 获取数据列表
* @return 数据列表
*/
@GetMapping(value = "/list")
List<Point> getList();
}
package cn.chendd.api.config;
import ;
/**
* 特定功能设置,不需要@Configuration注解
*
* @author chendd
* @date 2023/1/14 17:01
*/
public class FeatiresFeignConfiguration implements RequestInterceptor {
/**
* 自定义日志输出级别
* @return 日志输出
*/
@Bean
public Logger.Level loggerLevel() {
return Logger.Level.FULL;
}
/**
* 拦截器
* @param requestTemplate requestTemplate
*/
@Override
public void apply(RequestTemplate requestTemplate) {
//增加parameter参数
requestTemplate.query("name" , "chendd");
//增加header参数
requestTemplate.header("email" , "88911006@qq.com");
//覆盖url地址,可用于动态获取的url路径,也可以随便写个不存在的地址模拟接口调用失败,验证接口熔断
///requestTemplate.target("http://127.0.0.1:8020/");
//request请求参数获取
/*ServletRequestAttributes attributes = (ServletRequestAttributes) RequestContextHolder.getRequestAttributes();
if (attributes != null) {
HttpServletRequest request = attributes.getRequest();
if (request != null) {
requestTemplate.header("contextPath" , request.getContextPath());
}
}*/
}
}
/**
* OpenFeignFeaturesClient 降级
*
* @author chendd
* @date 2023/1/14 22:16
*/
@Component
public class OpenFeignFeaturesClientFallback implements OpenFeignFeaturesClient {
@Override
public List<Point> getList() {
return Lists.newArrayList();
}
}
/**
* OpenFeignFeaturesClient错误处理工厂
*
* @author chendd
* @date 2023/1/14 21:39
*/
@Component
public class OpenFeignFeaturesClientFallbackFactory implements FallbackFactory<OpenFeignFeaturesClient> {
@Override
public OpenFeignFeaturesClient create(Throwable cause) {
OpenFeginLogger.getLogger().error("调用接口出现错误:" + cause.getMessage() , cause);
return new OpenFeignFeaturesClient() {
//实际应用中此处应该是返回统一json数据结构,并且设置状态码为error类
@Override
public List<Point> getList() {
return Lists.newArrayList();
}
};
}
}
(1)OpenFeignFeaturesClient可以单独指定configuration,也可以在EnableFeignClients中全局指定;
(2)对于服务容错有fallback和fallbackFactory 两个参数,实际应用中推荐使用后者,后者可以获取异常堆栈;
(3)通过增加@Bean的形式增加Logger.Level组件来配置日志输出范围的级别;
(4)FeatiresFeignConfiguration不需要@Configuration注解;
(5)实现RequestInterceptor接口来增加请求拦截器,示例中有增加query请求参数、header请求头、target函数可以动态设置请求url路径(覆盖通过注解配置的部分);
(6)取消上述代码中的FeatiresFeignConfiguration.apply中的注释行requestTemplate.target,随便写个不存在的服务地址模拟程序报错可触发服务熔断回调;
(6)Open Feign的客户端调用传递对象前无法传递数据,需要把一个对象的多个参数给拆开,等于说客户端传递时分散传递,在controller端仍然使用对象接收;
(7)Open Feign的客户端传递String[]时传递到controller服务端时拿到的是String[]的地址,并非是具体的数据,后将String[]替换为List<String>可正常接收;
点赞